Extended Kalman Filter in Orekit
Covariance/noise matrix 在 body frame 和 inertial frame 之间的转换
这部分代码出现在 UnivariateProcessNoise
中,see
#537: `FIXME` in codes
#403: Add a provider generating process noise increasing in time, for better Kalman filtering
for more discussions.
RSW === LVLH in Orekit.
lofCartesianProcessNoiseMatrix
Local orbital frame (RSW, or LVLH) 中的 covariance
- GP-prediction covariance, a diagonal 6 x 6 matrix. (in my codes)
jacLofToInertial
- Question? Is there a loss of information at this step?
jacParametersWrtCartesian
- If the parameters used to represent the orbit is inertial Cartesian, then this should be .
- Otherwise, it’s not.
Finial conversion:
inverse: from Inertial to RSW
Simple:
代码细节
总结:
- 在 Hipparchus 中,需要估计的动力学模型被称为 process (
NonLinearProcess
);在 Orekit 中,Model
即是这个 process。 - 在 Hipparchus 中, estimator 计算过程中的结果称为
ProcessEstimate
, process 的计算结果称为 evolution (NonLinearEvolution.java
);在 Orekit 中,estimator 的计算结果称为(被我修改为KalmanEstimation
KalmanEstimate
,其它地方全是这么叫的,用 estimation 混乱。) [TODO: feed back to Orekit] - Hipparchus 只处理 normalized values, Orekit 通过
Model
来实现 dimensional value 和 normalized value 之前的交互。 - Hipparchus 只需要调用
Model
中的getEvolution()
和getInnovation()
;Model
中的所有其它内容,全部是处理 dynamics, normalizing/unnormalizing… - 想要的 EKF 的结果直接从 Orekit 的
KalmanEstimator
中找相应函数,基本都是Model
中对应函数的 wrapper。新功能在通过修改KalmanEstimator
来实现好过修改Model
(因为后者是 package private 的包,将来可能有很大的改动)。
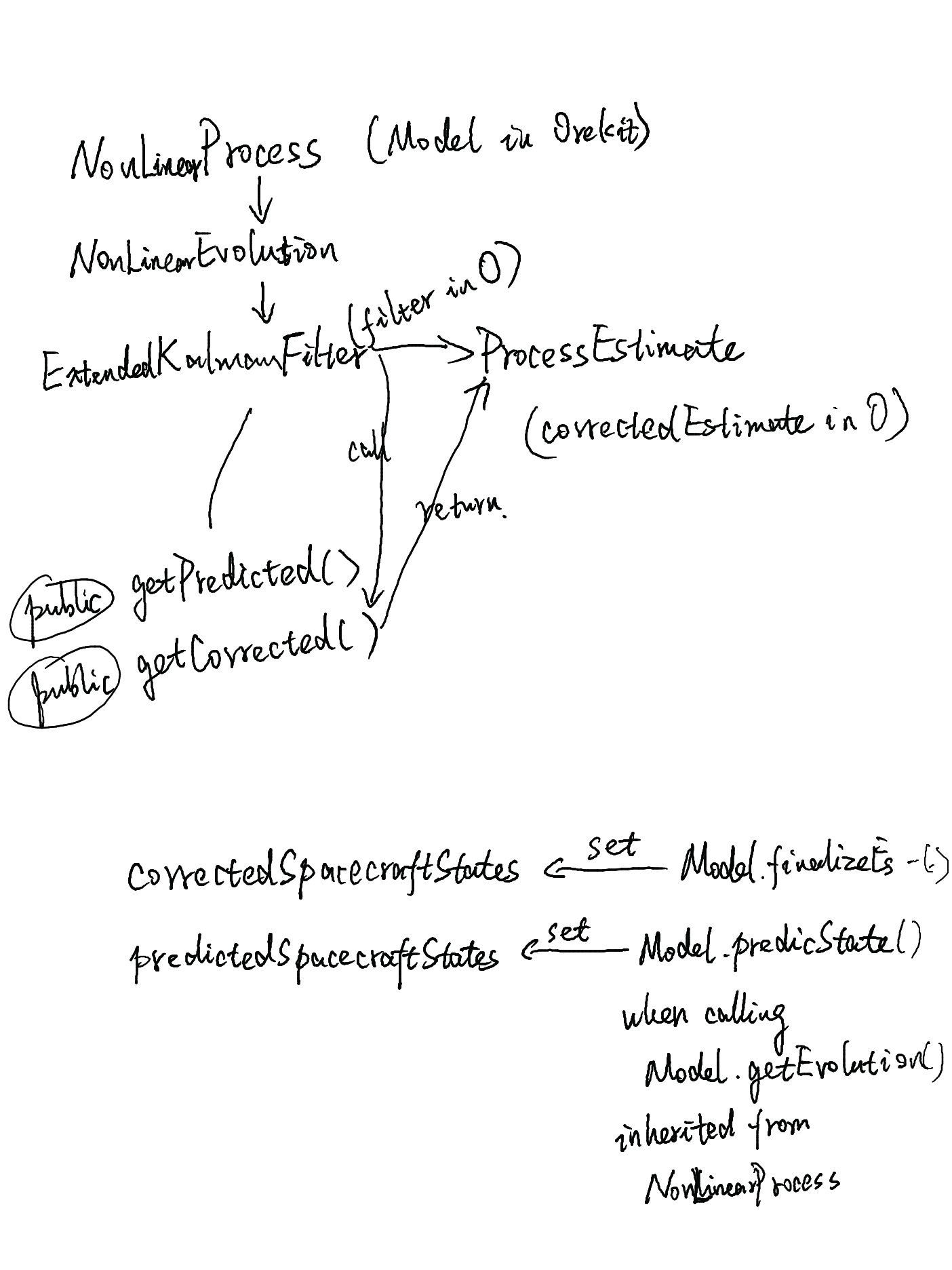
Where each values are stored
ExtendedKalmanFilter
实现了 AbstractKalmanFilter
实现了 KalmanFilter
(单步更新,然后读取预测和更新的结果)。
Orekit.*.Model
实现了 Hipparchus.*.NonLinearProcess
。
计算结果存在为 Hipparchus.*.NonLinearEvolution
。
Orekit.*.KalmanEstimator.estimateStep
中调用 filter.estimationStep()
返回的是 Hipparchus.*.ProcessEstimate
,包含了, , , , , , 。
Process noise
UnivariateProcessNoise
接受输入为:
初始误差initialCovarianceMatrix
,
RSW系轨道参数噪声函数lofCartesianOrbitalParametersEvolution
,
模型过程噪声函数propagationParametersEvolution
。
Get predicted covariance (with/without noise) at every epochs without duplicate propagation
AbstractKalmanFilter.java
中的 correct()
中可以利用下面这段代码,跳过当前 measurement 导致的更新过程,可以实现连续预测,在每个 epoch 得到相应的 predictedState, predictedCovariance, noise
1 | if (innovation == null) { |
Problems !
The covariance input used to construct
之前理解错误,是保留了PV
measurement is not kept by the constructor!!!
It is replaced by extracting sigma, i.e. square root of the diagonal of the covariance.
Orekit should mention this at some place (TODO: check and feedback).covariance
的,在AbstractMeasurement
中只保存sigma
,但是PV
保存了covariance
。
General Orekit
Hybrid Kalman Filter
和这个概念更接近,参见 Wikipedia: Kalman_filter#Hybrid_Kalman_filter。
Discrete-time measurements
或者是这个概念,参见 Wikipedia: Discrete-time_measurements。
Porpagator/Propagation paramerters 即是模型参数
See this Orekit architecture description:
- one list containing the 6 orbital parameters, which are estimated by default
- one list containing the propagator parameters, which depends on the force models used and are not estimated by default
- one list containing the measurements parameters, which are not estimated by default
对 process noise 的实现
积分state
时使用,没有process noise。
积分state transition matrix时使用,同样没有process noise。
最后计算covariance
时需要添加process noise ,可以假设是依赖于时间的。
General EKF
Equations
from Wikipedia: Discrete-time predict and update equations
Prediction:
Predicted state estimate
Predicted covariance estimate
Update:
Innovation or measurement residual
Innovation (or residual) covariance
Near-optimal Kalman gain
Updated state estimate
Updated covariance estimate
实际上更应该使用 Continuous-time extended Kalman filter with discrete-time measurements,公式都差不多。
In my study,
如果 , ,
则
则
是否
?
问题:
总是要“小于” 预测的不确定性 和观测误差 ?
当 和 其中之一远大于另一个时, 完成退化为较小的一个。
Testing results:
- force : test the conversion from inertial to RSW; covariance should be identical to EKF pure. [checked, correct.]
- force : test the conversion from RSW to inertial; covariance should be identical to GP pure. [checked, correct.]
- force equals : test the updating process; covariance should be half of them while standard deviation should be of them. [checked, correct.]
So, all calculations are correct.